Using Playroom with Cocos Creator
Cocos Creator (opens in a new tab) is a 2D/3D game engine that supports JavaScript and TypeScript. You can use Playroom with Cocos Creator to build multiplayer games.
Setup
Follow these steps to import Playroom into your project.
-
Create a new project in Cocos Creator.
-
Get a multiplayer.full.umd.js (opens in a new tab) and types.d.ts (opens in a new tab) (rename to playroom.d.ts for visibility) file into your project (newer versions of Cocos Creator default to TypeScript).
-
Import the multiplayer.full.umd.js as a plugin
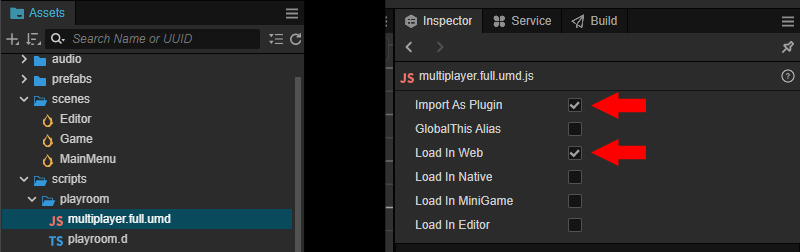
- Now you can start using Playroom in your Cocos Creator scripts like this:
import * as PlayroomType from './playroom.d.ts';
const Playroom: typeof PlayroomType = window.Playroom;
export class MyScript extends Component {
async start() {
await Playroom.insertCoin({
// any options here
});
}
}